When you're trying to migrate an application from `pages/` to `app/`,
you'll need to access data like search parameters and the pathname in a
way that lets you migrate safely.
This adds support for dynamic typing of some of those exported functions
from `next/navigation`, namely `useSearchParams` and `usePathname`.
Currently, `searchParams` can’t be known when prerendering if the page
doesn’t use [Server-side
Rendering](https://nextjs.org/docs/basic-features/data-fetching/get-server-side-props)
in the `pages/` directory. `pathname` can’t be known during prerendering
if the page is a fallback page or has been automatically statically
optimized when accessed from `pages/`.
To make migraitons easier, this adds a new feature to `next dev` that
will automatically add the correct types for `next/navigation`. It does
this by checking if you have both a `app/` and `pages/` directory. If it
detects you have a `app/` directory, it will also enable the suggested
Typescript feature,
[`structNullChecks`](https://www.typescriptlang.org/tsconfig#strictNullChecks)
which will warn developers when trying to access a value that may be
`null`.
---------
Co-authored-by: JJ Kasper <jj@jjsweb.site>
After speaking with @timneutkens, this PR provides a smoother experience to users trying to migrate over to app without affecting users in pages.
This PR adds a new export available from `next/compat/router` that exposes a `useRouter()` hook that can be used in both `app/` and `pages/`. It differs from `next/router` in that it does not throw an error when the pages router is not mounted, and instead has a return type of `NextRouter | null`. This allows developers to convert components to support running in both `app/` and `pages/` as they are transitioning over to `app/`.
A component that before looked like this:
```tsx
import { useRouter } from 'next/router';
const MyComponent = () => {
const { isReady, query } = useRouter();
// ...
};
```
Will error when converted over to `next/compat/router`, as `null` cannot be destructured. Instead, developers will be able to take advantage of new hooks:
```tsx
import { useEffect } from 'react';
import { useRouter } from 'next/compat/router';
import { useSearchParams } from 'next/navigation';
const MyComponent = () => {
const router = useRouter() // may be null or a NextRouter instance
const searchParams = useSearchParams()
useEffect(() => {
if (router && !router.isReady) {
return
}
// In `app/`, searchParams will be ready immediately with the values, in
// `pages/` it will be available after the router is ready.
const search = searchParams.get('search')
// ...
}, [router, searchParams])
// ...
}
```
This component will now work in both `pages/` and `app/`. When the component is no longer used in `pages/`, you can remove the references to the compat router:
```tsx
import { useSearchParams } from 'next/navigation';
const MyComponent = () => {
const searchParams = useSearchParams()
// As this component is only used in `app/`, the compat router can be removed.
const search = searchParams.get('search')
// ...
}
```
Note that as of Next.js 13, calling `useRouter` from `next/router` will throw an error when not mounted. This now includes an error page that can be used to assist developers.
We hope to introduce a codemod that can convert instances of your `useRouter` from `next/router` to `next/compat/router` in the future.
Co-authored-by: JJ Kasper <22380829+ijjk@users.noreply.github.com>
This PR introduces breaking changes by renaming components.
- Rename `next/image` to `next/legacy/image`
- Rename `next/future/image` to `next/image`
The diff is very confusing because both components are very similar so git got confused.
I noticed our internal comments about exported functions that should not be used in application code.
TSDoc has the [`@internal`](https://tsdoc.org/pages/tags/internal/) option that can be used together with TS's [`stripInternal: true` compiler option](https://www.typescriptlang.org/tsconfig/#stripInternal), hiding these from inferred types:
Before:
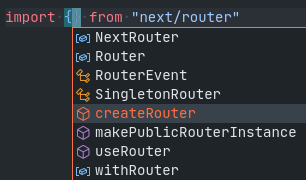
After:
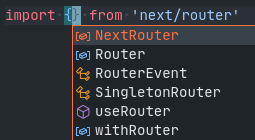
It would also be great to use `/**/` comments instead of `//` when documenting methods/properties/functions, as these can be picked up by the editor when referring to these, so someone new to the codebase will hopefully have to jump around less to get some useful comments.
## Bug
- [ ] Related issues linked using `fixes #number`
- [ ] Integration tests added
- [ ] Errors have helpful link attached, see `contributing.md`
## Feature
- [ ] Implements an existing feature request or RFC. Make sure the feature request has been accepted for implementation before opening a PR.
- [ ] Related issues linked using `fixes #number`
- [ ] Integration tests added
- [ ] Documentation added
- [ ] Telemetry added. In case of a feature if it's used or not.
- [ ] Errors have helpful link attached, see `contributing.md`
## Documentation / Examples
- [ ] Make sure the linting passes by running `pnpm lint`
- [ ] The examples guidelines are followed from [our contributing doc](https://github.com/vercel/next.js/blob/canary/contributing.md#adding-examples)
This PR introduces a new experimental component, `next/future/image`, which is inspired by the existing experimental `layout="raw"`.
The difference is that much of the code has been deleted in order to reduce client-side code as well as reduce complexity:
- No `layout` prop
- No `loader` config (although `loader` prop works)
- No `IntersectionObserver`, use native `loading="lazy"`
- No `lazyBoundary`
- No `lazyRoot`
- No `fill` (yet) so width & height are required
- No `objectFit` (use `style` instead)
- No `objectPosition` (use `style` instead)
This improves performance because native `loading="lazy"` doesn't need to wait for React Hydration and client-side JS.
In a future PR, we will modify `next/image` to remove `layout="raw"` since this new component supersedes it.
## Feature
- [x] Integration tests added
- [x] Documentation added
- [x] Telemetry added. In case of a feature if it's used or not.
- [x] Errors have helpful link attached, see `contributing.md`
* Move type to image component
* Add types/global.d.ts to excludes too
* Undo global exclude as it's using internally
* Don't add root imports for module augmentations
* Run prettier over packages/**/*.js
* Run prettier over packages/**/*.ts
* Run prettier over examples
* Remove tslint
* Run prettier over examples
* Run prettier over all markdown files
* Run prettier over json files
- Replaces taskr-babel with taskr-typescript for the `next` package
- Makes sure Node 8+ is used, no unneeded transpilation
- Compile Next.js client side files through babel the same way pages are
- Compile Next.js client side files to esmodules, not commonjs, so that tree shaking works.
- Move error-debug.js out of next-server as it's only used/require in development
- Drop ansi-html as dependency from next-server
- Make next/link esmodule (for tree-shaking)
- Make next/router esmodule (for tree-shaking)
- add typescript compilation to next-server
- Remove last remains of Flow
- Move hoist-non-react-statics to next, out of next-server
- Move htmlescape to next, out of next-server
- Remove runtime-corejs2 from next-server